Carousel - Type A
This is a Carousel component. Carousel allows us to show multiple images in the same space. Its can be used when:-
- There is a group of content on the same level.
- There is insufficient content space, it can be used to save space.
- Commonly used for a group of pictures/cards.
[Note]:- There is also another type of carousel component called CarouselTypeB component.
Props
Props | Type | Required | Description |
---|---|---|---|
images | Array<string> | Yes | A string array containing the addresses of all the carousel images |
rotate | boolean | No | When |
UserIndicatorComponent | React.FC<UserIndicator ComponentProps> | No | User can pass this component to replace the default indicator |
UserNavigationButtons | React.FC<UserNavigation ButtonsProps> | No | User can pass this component to replace the default left and right |
UserIndicatorComponentProps
The UserIndicatorComponent
renders a single dot and is mapped over the images array to render the corresponding dot for each image. It should accept the follwing props.
Props | Type | Required | Description |
---|---|---|---|
index | number | Yes | The index of the current image in the array |
activeIndex | number | Yes | The index of the current selected image in the array |
changeImage | (index: number) => void | Yes | A function that gets called on clicking a dot. When you click a dot, its corresponding index is set as the activeIndex |
UserNavigationButtonsProps
The UserNavigationButtons
can be used to pass a user navigation component. It should accept the following props.
Props | Type | Required | Description |
---|---|---|---|
slideLeft | ( ) => void | Yes | A function that will be passed to enable the left navigation in the carousel |
slideRight | ( ) => void | Yes | A function that will be passed to enable the right navigation in the carousel |
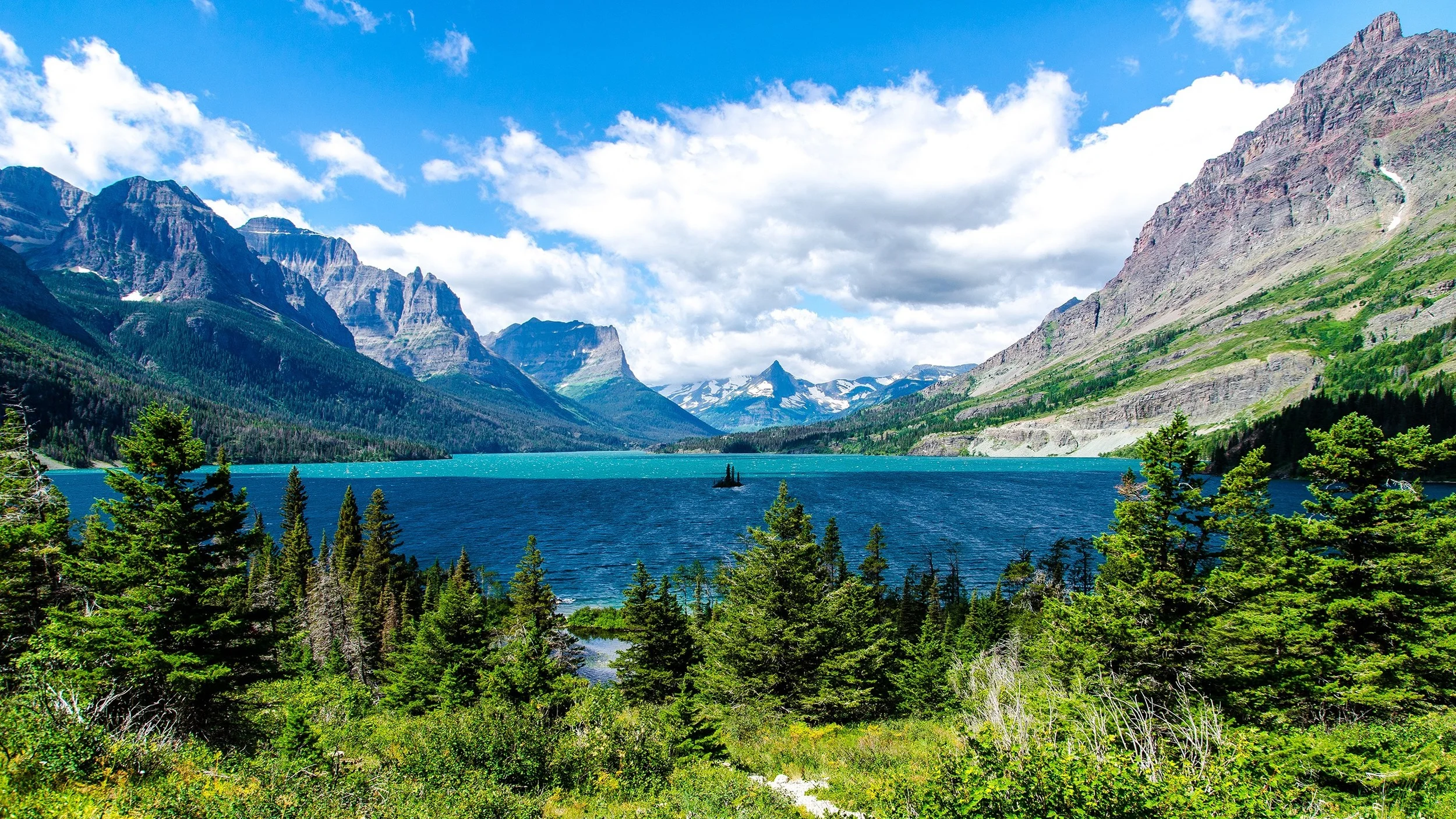
import {
CarouselTypeA,
UserIndicatorComponent,
UserNavigationButtons,
} from "canary-design";
const images = [
"https://images.squarespace-cdn.com/content/v1/57afe07729687fbd6a7971ad/1471718394640-AYK8C3POPTKL2GU5DGRM/Glacier.National.Park.original.494.jpg?format=2500w",
"https://dynamic-media-cdn.tripadvisor.com/media/photo-o/17/f3/ba/50/royal-arches-on-the-left.jpg?w=1100&h=-1&s=1",
"https://cdn.britannica.com/46/193946-050-853B37E0/Yosemite-National-Park-California.jpg",
"https://res.klook.com/images/fl_lossy.progressive,q_65/c_fill,w_1170,h_780/w_72,x_13,y_13,g_south_west,l_Klook_water_br_trans_yhcmh3/activities/rcoclkcqcpyoogx1rbvl/4-DayYellowstoneNationalParkLuxuryTourfromSaltLakeCity.jpg",
"https://lp-cms-production.imgix.net/2021-03/shutterstockRF_1180791211.jpg",
];
function ExampleCarouselTypeA() {
return (
/* Note:- The carousel will automatically take the entire width and height of the parent. We have given fixed height to parent because we want all images to appear of same height. */
<div style={{ height: "384px" }}>
<CarouselTypeA
images={images}
rotate
/* the UserIndicatorComponent and the UserNavigationButtons are optional components */
UserIndicatorComponent={(props) => (
<UserIndicatorComponent {...props} />
)}
UserNavigationButtons={(props) => <UserNavigationButtons {...props} />}
/>
</div>
);
}
export { ExampleCarouselTypeA };
UserIndicatorComponent
import styles from "src/components/UserIndicatorComponent/index.module.css";
interface UserIndicatorComponentProps {
index: number;
activeIndex: number;
changeImage: (index: number) => void;
}
const UserIndicatorComponent = ({
index,
activeIndex,
changeImage,
}: UserIndicatorComponentProps) => {
return (
<div className={styles["dot"]} onClick={() => changeImage(index)}>
{index === activeIndex && <div className={styles["inner-dot"]}></div>}
</div>
);
};
.dot {
height: 15px;
width: 15px;
background-color: #efefef;
border-radius: 20%;
cursor: pointer;
padding: 2px;
}
.inner-dot {
height: 100%;
width: 100%;
border-radius: 20%;
background-color: grey;
}
UserNavigationButtons
import styles from "src/components/UserNavigationButtons/index.module.css";
import leftIconBlack from "src/assets/caret-left-black.svg";
import rightIconBlack from "src/assets/caret-right-black.svg";
import leftIconWhite from "src/assets/caret-left-white.png";
import rightIconWhite from "src/assets/caret-right-white.png";
interface UserNavigationButtonsProps {
slideLeft: () => void;
slideRight: () => void;
}
function UserNavigationButtons({
slideLeft,
slideRight,
}: UserNavigationButtonsProps) {
return (
<div className={styles["navigation-buttons"]}>
<div className={styles["nav-button"]} onClick={slideLeft}>
<div className={styles["icon-container"]}>
<img src={leftIconWhite} />
</div>
</div>
<div className={styles["nav-button"]} onClick={slideRight}>
<div className={styles["icon-container"]}>
<img src={rightIconWhite} />
</div>
</div>
</div>
);
}
.navigation-buttons {
display: flex;
justify-content: space-between;
padding: 2px 8px;
position: absolute;
top: 50%;
width: 100%;
font-weight: 600;
color: #fff;
cursor: pointer;
box-sizing: border-box;
}
.nav-button {
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
}
.icon-container {
height: 24px;
width: 24px;
}
.icon-container img {
height: 100%;
width: 100%;
}